DM Lab¶
DeepMind Lab¶
DM Lab is a suite of challenging 3D navigation and puzzle-solving tasks for learning agents, based on id Software’s Quake III Arena via ioquake3 and other open source software.
Shimmy provides compatibility wrappers to convert all DM Lab environments to Gymnasium.
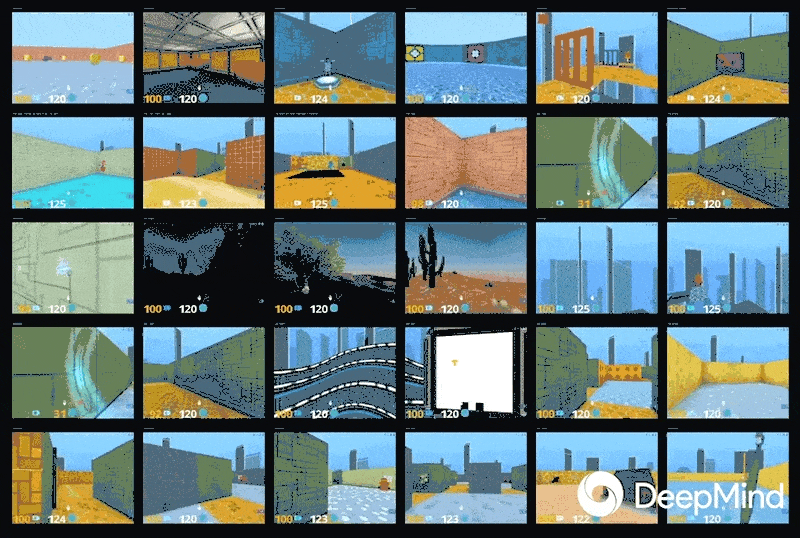
Installation¶
pip install shimmy[dm-lab]
DeepMind Lab is not distributed via pypi and must be installed manually. Courtesy to Danijar Hafner for providing an install script. For troubleshooting, refer to the official installation instructions.
We also provide a Dockerfile for reproducibility and cross-platform compatibility:
curl https://raw.githubusercontent.com/Farama-Foundation/Shimmy/main/bin/dm_lab.Dockerfile | docker build -t dm_lab -f - . && docker run -it dm_lab
Warning
DeepMind Lab does not currently support Windows or macOS operating systems.
Usage¶
Load a deepmind_lab
environment:
import deepmind_lab
from shimmy.dm_lab_compatibility import DmLabCompatibilityV0
observations = ["RGBD"]
config = {"width": "640", "height": "480", "botCount": "2"}
renderer = "hardware"
env = deepmind_lab.Lab("lt_chasm", observations, config=config, renderer=renderer)
env = DmLabCompatibilityV0(env)
Run the environment:
observation, info = env.reset()
for _ in range(1000):
action = env.action_space.sample() # this is where you would insert your policy
observation, reward, terminated, truncated, info = env.step(action)
if terminated or truncated:
observation, info = env.reset()
env.close()
Warning
Using gym.make() to load DM Lab environments is not currently supported.
We provide a helper function to load DM Lab environments, but cannot guarantee compatibility with all configurations. For troubleshooting, see Python API.
Class Description¶
- class shimmy.dm_lab_compatibility.DmLabCompatibilityV0(env: Any | None = None, level_name: str | None = None, observations: str | None = None, renderer: str | None = None, width: int | None = None, height: int | None = None, fps: int | None = None, mixerSeed: int | None = None, levelDirectory: str | None = None, appendCommand: str | None = None, botCount: int | None = None, render_mode: None = None)[source]¶
This compatibility wrapper converts a DM Lab environment into a gymnasium environment.
DeepMind Lab is a 3D learning environment based on id Software’s Quake III Arena via ioquake3 and other open source software. DeepMind Lab provides a suite of challenging 3D navigation and puzzle-solving tasks for learning agents. Its primary purpose is to act as a testbed for research in artificial intelligence, especially deep reinforcement learning.
Wrapper to convert a DM Lab environment into a Gymnasium environment.
Note: to wrap an existing environment, only the env and render_mode arguments can be specified. All other arguments are specific to DM Lab and will be used to load a new environment.
- Parameters:
env (Optional[Any]) – existing DM Lab environment to wrap
level_name (Optional[str]) – name of level to load
observations – (Optional[str]): type of observations to use (default: “RGBD”)
renderer (Optional[str]) – renderer to use (default: “hardware”)
width (Optional[int]) – horizontal resolution of the observation frames (default: 240)
height (Optional[int]) – vertical resolution of the observation frames (default: 320)
fps (Optional[int]) – frames-per-second (default: 60)
mixerSeed (Optional[int]) – value combined with each of the seeds fed to the environment to define unique subsets of seeds (default: 0)
levelDirectory (Optional[str]) – optional path to level directory (relative paths are relative to game_scripts/levels)
appendCommand (Optional[str]) – Commands for the internal Quake console
botCount (Optional[int]) – number of bots to use
render_mode (Optional[str]) – rendering mode to use (choices: “human”, “none”
- metadata: dict[str, Any] = {'render_fps': 10, 'render_modes': ['human']}¶
- reset(*, seed: int | None = None, options: dict[str, Any] | None = None) tuple[ObsType, dict[str, Any]] [source]¶
Resets the dm-lab environment.
Utils¶
Utility functions for the compatibility wrappers.
- shimmy.utils.dm_lab.load_dm_lab(level_name: str = 'lt_chasm', observations: str | None = 'RGBD', renderer: str | None = 'hardware', width: int | None = 320, height: int | None = 240, fps: int | None = 60, mixerSeed: int | None = 0, levelDirectory: str | None = '', appendCommand: str | None = '', botCount: int | None = None)[source]¶
Helper function to load a DM Lab environment.
Handles arguments which are None or unspecified (which will throw errors otherwise).
- Parameters:
level_name (str) – name of level to load
observations – (Optional[str]): type of observations to use (default: “RGBD”)
renderer (Optional[str]) – renderer to use (default: “hardware”)
width (Optional[int]) – horizontal resolution of the observation frames (default: 240)
height (Optional[int]) – vertical resolution of the observation frames (default: 320)
fps (Optional[int]) – frames-per-second (default: 60)
mixerSeed (Optional[int]) – value combined with each of the seeds fed to the environment to define unique subsets of seeds (default: 0)
levelDirectory (Optional[str]) – optional path to level directory (relative paths are relative to game_scripts/levels)
appendCommand (Optional[str]) – Commands for the internal Quake console
botCount (Optional[int]) – number of bots to use
- Returns:
env – DM Lab environment