OpenSpiel¶
DeepMind OpenSpiel¶
OpenSpiel is a collection of 70+ environments for common board games, card games, as well as simple grid worlds and social dilemmas.
It supports n-player (single- and multi- agent) zero-sum, cooperative and general-sum, one-shot and sequential, strictly turn-taking and simultaneous-move, perfect and imperfect information games.
Shimmy provides compatibility wrappers to convert all OpenSpiel environments to PettingZoo.
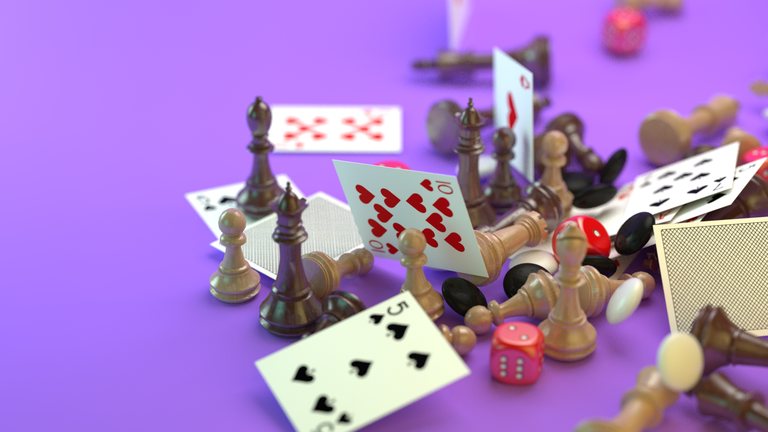
Note: PettingZoo also provides popular board & card game environments: PettingZoo Classic.
Installation¶
To install shimmy
and required dependencies:
pip install shimmy[openspiel]
We also provide a Dockerfile for reproducibility and cross-platform compatibility:
curl https://raw.githubusercontent.com/Farama-Foundation/Shimmy/main/bin/openspiel.Dockerfile | docker build -t openspiel -f - . && docker run -it openspiel
Warning
OpenSpiel does not currently support Windows operating systems.
Usage¶
Load an openspiel
environment:
from shimmy import OpenSpielCompatibilityV0
env = OpenSpielCompatibilityV0(game_name="backgammon", render_mode="human")
Wrap an existing openspiel
environment:
import pyspiel
from shimmy.openspiel_compatibility import OpenSpielCompatibilityV0
env = pyspiel.load_game("2048")
env = OpenSpielCompatibilityV0(env)
Note: using the env
and game_name
arguments together will result in a ValueError
.
Use
env
to wrap an existing OpenSpiel environment.Use
game_name
to load a new OpenSpiel environment.
Run the environment:
env.reset()
for agent in env.agent_iter():
observation, reward, termination, truncation, info = env.last()
if termination or truncation:
action = None
else:
action = env.action_space(agent).sample(info["action_mask"]) # this is where you would insert your policy
env.step(action)
env.render()
env.close()
Warning
OpenSpiel does not support random seeding for all environments.
Warning
OpenSpiel does not support graphical rendering, and only supports ASCII text rendering for some environments. Environments which do not provide rendering will print the internal game state.
Class Description¶
- class shimmy.openspiel_compatibility.OpenSpielCompatibilityV0(env: pyspiel.Game | None = None, game_name: str | None = None, render_mode: str | None = None, config: dict | None = None)[source]¶
This compatibility wrapper converts an OpenSpiel environment into a PettingZoo environment.
OpenSpiel is a collection of environments and algorithms for research in general reinforcement learning and search/planning in games. OpenSpiel supports n-player (single- and multi- agent) zero-sum, cooperative and general-sum, one-shot and sequential, strictly turn-taking and simultaneous-move, perfect and imperfect information games, as well as traditional multiagent environments such as (partially- and fully- observable) grid worlds and social dilemmas.
Wrapper to convert a OpenSpiel environment into a PettingZoo environment.
- Parameters:
env (Optional[pyspiel.Game]) – existing OpenSpiel environment to wrap
game_name (Optional[str]) – name of OpenSpiel game to load
render_mode (Optional[str]) – rendering mode
config (Optional[dict]) – PySpiel config
- metadata: dict[str, Any] = {'is_parallelizable': False, 'name': 'OpenSpielCompatibilityV0', 'render_modes': ['human']}¶
- observation_space(agent: AgentID)[source]¶
observation_space.
We get the observation space from the underlying game. OpenSpiel possibly provides information and observation in several forms. This wrapper chooses which one to use depending on the following precedence: 1. Observation Tensor 2. Information Tensor 3. Observation String 4. Information String
- Parameters:
agent (AgentID) – agent
- Returns:
space (gymnasium.spaces.Space) – observation space for the specified agent
- action_space(agent: AgentID)[source]¶
action_space.
Get the action space from the underlying OpenSpiel game.
- Parameters:
agent (AgentID) – agent
- Returns:
space (gymnasium.spaces.Space) – action space for the specified agent
- observe(agent: AgentID) Any [source]¶
observe.
- Parameters:
agent (AgentID) – agent
- Returns:
observation (Any)