Behavior Suite¶
DeepMind Behavior Suite¶
Behavior Suite is a collection of carefully-designed experiments that investigate various aspects of agent behavior through shared benchmarks.
Shimmy provides compatibility wrappers to convert Behavior Suite environments to Gymnasium.
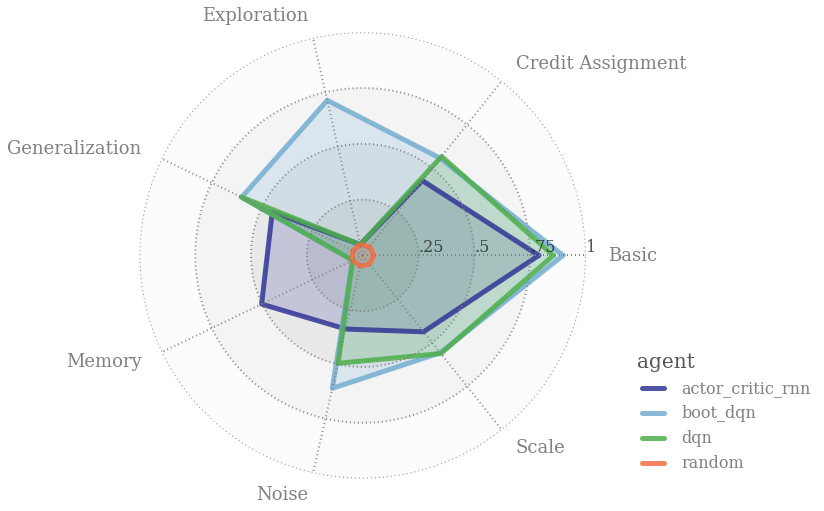
Installation¶
To install shimmy
and required dependencies:
pip install shimmy[bsuite]
We also provide a Dockerfile for reproducibility and cross-platform compatibility:
curl https://raw.githubusercontent.com/Farama-Foundation/Shimmy/main/bin/bsuite.Dockerfile | docker build -t bsuite -f - . && docker run -it bsuite
Usage¶
Load a bsuite
environment:
import gymnasium as gym
env = gym.make("bsuite/catch-v0", render_mode="human")
Run the environment:
observation, info = env.reset(seed=42)
for _ in range(1000):
action = env.action_space.sample() # this is where you would insert your policy
observation, reward, terminated, truncated, info = env.step(action)
if terminated or truncated:
observation, info = env.reset()
env.close()
To get a list of all available bsuite
environments (23 total):
from gymnasium.envs.registration import registry
BSUITE_ENV_IDS = [
env_id
for env_id in registry
if env_id.startswith("bsuite") and env_id != "bsuite/compatibility-env-v0"
]
print(BSUITE_ENV_IDS)
Class Description¶
- class shimmy.bsuite_compatibility.BSuiteCompatibilityV0(env: Environment, render_mode: str | None = None)[source]¶
A compatibility wrapper that converts a BSuite environment into a gymnasium environment.
Note
Bsuite uses np.random.RandomState, a legacy random number generator while gymnasium uses np.random.Generator, therefore the return type of np_random is different from expected.
Initialises the environment with a render mode along with render information.
- metadata: dict[str, Any] = {'render_modes': []}¶
- reset(*, seed: int | None = None, options: dict[str, Any] | None = None) tuple[ObsType, dict[str, Any]] [source]¶
Resets the bsuite environment.
- step(action: int) tuple[ObsType, float, bool, bool, dict[str, Any]] [source]¶
Steps through the bsuite environment.
- property np_random: RandomState¶
This should be np.random.Generator but bsuite uses np.random.RandomState.